UOMOP
Functional API 본문
- module 호출
- 함수 생성
- 데이터 불러오기
- 0~1 함수 적용
- one hot encoding
- 학습/검증/테스트 데이터 분리
- 모델 생성
- 모델 설정
- 모델 학습
- 학습/검증 셋으로 성능 비교
- 테스트 셋을 통한 성능 확인
1. module 호출
import matplotlib.pyplot as plt
import numpy as np
from sklearn.model_selection import train_test_split
from tensorflow.keras.utils import to_categorical
from tensorflow.keras.layers import Input, Flatten, Dense
from tensorflow.keras.models import Model
from tensorflow.keras.datasets import fashion_mnist
from tensorflow.keras.optimizers import Adam
2. 함수 생성
# 0~1로 변경
def make_zero_to_one(images, labels) :
scaled_images = np.array(images/255., dtype = np.float32)
scaled_labels = np.array(labels, dtype = np.float32)
return scaled_images, scaled_labels
# one-hot encoding
def ohe(labels) :
oh_labels = to_categorical(labels)
return oh_labels
# 데이터 분리
def seperate_3(train_images, train_labels, test_images, test_labels, val_rate) :
tr_images, val_images, tr_labels, val_labels = train_test_split(train_images,
train_labels, test_size = val_rate)
return (tr_images, tr_labels), (val_images, val_labels), (test_images, test_labels)
# Functional API 모델 생성하기
def create_model(input_data) :
input_size = (input_data[0, :, :].shape)[0]
input_tensor = Input(shape = (input_size, input_size))
x = Flatten()(input_tensor)
x = Dense(100, activation = "relu")(x)
x = Dense(30, activation = "relu")(x)
output = Dense(10, activation = "softmax")(x)
model = Model(inputs = input_tensor, outputs = output)
return model
3. 데이터 불러오기
(train_images, train_labels), (test_images, test_labels) = fashion_mnist.load_data()
4. 함수 적용
# 0~1
train_images, train_labels = make_zero_to_one(train_images, train_labels)
test_images, test_labels = make_zero_to_one(test_images, test_labels)
# one-hot encoding
train_labels = ohe(train_labels)
test_labels = ohe(test_labels)
# 데이터 분리
(tr_images, tr_labels),
(val_images, val_labels),
(test_images, test_labels) = seperate_3(train_images, train_labels, test_images, test_labels,
val_rate = 0.15)
5. 모델 생성
model = create_model(tr_images)
model.summary()
6. 모델 설정 (최적화(학습률), 손실함수, 성능지표)
model.compile(optimizer = Adam(0.001), loss = "categorical_crossentropy", metrics = ["accuracy"])
7. 모델 학습
result = model.fit(x = tr_images, y = tr_labels, batch_size = 32, epochs = 20,
validation_data = (val_images, val_labels))
8. 학습/검증 셋으로 성능 비교
fig, axs = plt.subplots(nrows = 1, ncols = 2, figsize = (10, 3))
axs[0].plot(result.history["accuracy"], label = "tr_acc", color = "r")
axs[0].plot(result.history["val_accuracy"], label = "val_acc", color = "b")
axs[0].set_xlabel("epochs")
axs[0].set_ylabel("accuracy")
axs[0].legend()
axs[0].set_title("compare accuracy")
axs[1].plot(result.history["loss"], label = "tr_loss", color = "r")
axs[1].plot(result.history["val_loss"], label = "val_loss", color = "b")
axs[1].set_xlabel("epochs")
axs[1].set_ylabel("loss")
axs[1].legend()
axs[1].set_title("compare loss")
9. 테스트 셋으로 성능 확인
eval = model.evaluate(test_images, test_labels, batch_size = 32, verbose = 1)
print("")
print("test data로 확인해본 model의 loss : {:.5f}".format(eval[0]))
print("test data로 확인해본 model의 accuracy : {:.5f}".format(eval[1]))
***one-hot encoding***
다중 분류를 위한 모델 학습을 하는 것이 목표이다. 이때 다중 분류를 위해서 출력단의 활성화 함수는 "softmax"이고 손실함수는 "categorical_crossentropy"이다.
만약, 손실함수가 "categorical_crossentropy"이면 label을 꼭 one-hot encoding 시켜야한다.
손실함수가 "sparse_categorical_crossentropy"이면 one-hot encoding 안해도 된다.
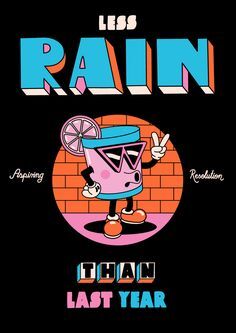
'Ai > DL' 카테고리의 다른 글
feature map size 계산 (0) | 2022.02.07 |
---|---|
CNN model 생성 (0) | 2022.02.06 |
미니배치 경사 하강법 using algorithm (boston) (0) | 2022.02.01 |
확률적 경사 하강법 using algorithm (boston) (0) | 2022.02.01 |
배치 경사 하강법 using keras (boston) (0) | 2022.02.01 |
Comments